Carousel
Overview
Grial's Carousel is used to display a list of elements in a similar fashion than .NET MAUI's CarouselView. It's very flexible, providing properties to control its looks, its behavior, and its animations. As an example, you can define a scaling factor for the previous and next element; and, when combined with the peek area looks like this:
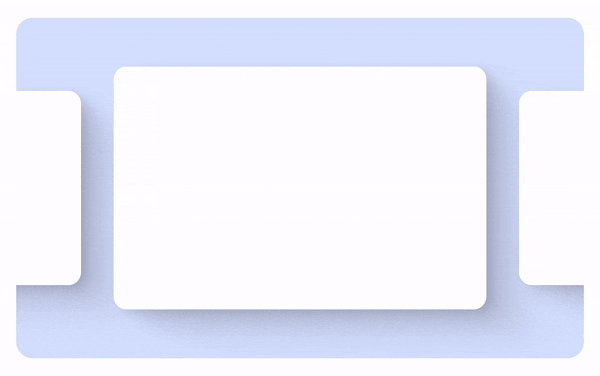
Animations: comes with two different pre-baked animations that differ on how the items scaling factors update while moving
Commands: MoveEndedCommand and PositionChangedCommand to notify ViewModels when the control did change; MoveNextCommand and MovePrevCommand to animate the carousel from a bound command
Mode-View-ViewModel ready: the content is defined through ItemsSource and ItemTemplate properties, and it provides a variety of commands and properties to pass the Carousel state to your ViewModels and control it from C# code
Optimized for performance: it’s 100% virtualized by default, it’s possible to opt out though
Flexible API: loop, position, item separation, selected item, enable/disable dragging, enable/disable position changes, events and differentiated item peek percentage and scale on each side
Progressive Animations integration: exposes ScrollProgress property which enables direct integration with Grial’s Progressive Animations
Indicator view support: can be linked to an IndicatorView in the same as MAUI’s CarouselView
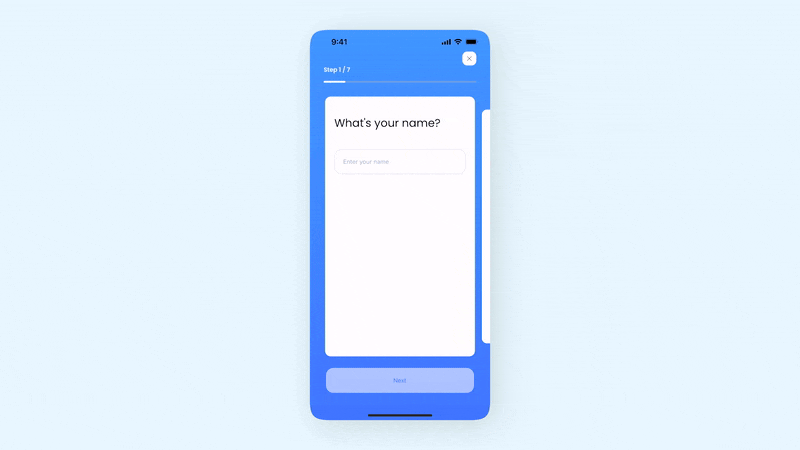
Properties
Name | Type | Description |
---|---|---|
Animation | AnimationType | Gets or sets the animation type from the the available predefined values. |
CachingStrategy | CachingStrategy | Gets or sets the strategy used for virtualization. |
CanMoveNext | bool | Gets or sets whether the control can move to the next position or not. |
CanMovePrev | bool | Gets or sets whether the control can move to the previous position or not. |
IndicatorView | IndicatorView | Gets or sets an IndicatorView instance to associate to the Carousel. |
IsDraggingEnabled | bool | Gets or sets whether drag/swipe user interaction is enabled or not. |
ItemSeparation | double | Gets or sets separation between items. |
ItemsSource | IEnumerable | Gets or sets the data collection used to populate the Carousel. |
ItemTemplate | DataTemplate | Gets or sets the DataTemplate used to display the items set in the ItemsSource. |
Loop | bool | Gets or sets whether the Carousel should loop or not. |
MoveEndedCommandParameter | object | Gets or sets the command parameter passed to MoveEndedCommand. If nothing is specified an instance of CarouselMovedEndedEventArgs is used. |
NextPeekPercentage | double | Gets or sets the percentage of the Carousel's area used to peek the next item. |
NextPeekScale | double | Gets or sets the scaling factor used to display the next item. |
PeekPercentage | double | Gets or sets the percentage of the Carousel's area used to peek the previous and next items. |
PeekScale | double | Gets or sets the scaling factor used to display the previous and next items. |
Position | int | Gets or sets the Position of the item currently displayed at the center. |
PositionChangedCommandParameter | object | Gets or sets the command parameter passed to PositionChangedCommand. If nothing is specified an instance of CarouselPositionChangedEventArgs is used. |
PrevPeekPercentage | double | Gets or sets the percentage of the Carousel's area used to peek the previous item. |
PrevPeekScale | double | Gets or sets the scaling factor used to display the previous item. |
ScrollProgress | double | Gets the a value indicating the change in the scroll while the Carousel is moving. The value is always between -1 and 1. |
SelectedItem | object | Gets or sets whether the selected item, i.e. the item currently displayed at the center. |
Events
Name | Type | Description |
---|---|---|
Moved | EventHandler<RelativeMoveEventArgs> | Event invoked while the Carousel is moving. |
MoveEnded | EventHandler<CarouselMovedEndedEventArgs> | Event invoked when the Carousel ends moving. |
PositionChanged | EventHandler<CarouselPositionChangedEventArgs> | Event invoked when the Carousel position changes. |
Commands
Name | Type | Description |
---|---|---|
MoveEndedCommand | ICommand | Gets or sets a command invoked when the Carousel stops moving. |
MoveNextCommand | ICommand | Gets a command invoked when the Carousel moves to the next position. |
MovePrevCommand | ICommand | Gets a command invoked when the Carousel moves to the previous position. |
PositionChangedCommand | ICommand | Gets or sets a command invoked when the Carousel's Position changes. |
Methods
Name | Type | Description |
---|---|---|
MoveNextAnimated(int duration = 200) | Task<bool> | Makes the Carousel move to the next element with the indicated animation duration. |
MovePrevAnimated(int duration = 200) | Task<bool> | Makes the Carousel move to the previous element with the indicated animation duration. |