Circular Slider
Overview
The Circular Slider control is a versatile UI component that allows users to select a value by rotating a handle along a circular path. This control is particularly useful for applications that require a rotary input method, such as setting a timer, selecting a volume level, or adjusting other parameters that usually are represented with a dial control.
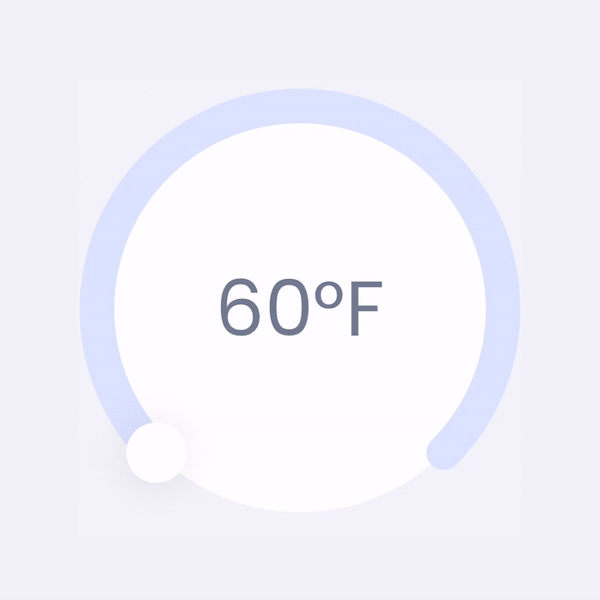
How to Use It
To use the Circular Slider control in your application, follow these steps:
XAML Usage
Add the Circular Slider control to your XAML page.
<grial:CircularSlider
x:Name="circularSlider"
Minimum="0"
Maximum="100"
Value="50"
TrackColor="LightGray"
ActiveColor="Blue"
ThumbColor="Red"
ValueChanged="OnCircularSliderValueChanged" />
Event Handling
Handle the ValueChanged event in your code-behind to respond to user input.
private void OnCircularSliderValueChanged(object sender, ValueChangedEventArgs e)
{
// Handle the value change
double newValue = e.NewValue;
// Update UI or perform other actions based on the new value
}
info
You can also use the commands DragCompletedCommand
, DragStartedCommand
, and ValueChangedCommand
, to interact with the control.
Control API
Properties
Name | Type | Description |
---|---|---|
ActiveColor | Color | Gets or sets the color of the arc between StartAngle and the angle representing the Value. |
ActiveRadiusDelta | double? | Gets or sets the active area radius offset respect to the inactive area radius. |
ActiveStrokeLineCap | LineCap | Gets or sets the stroke cap of the active area. |
ActiveThickness | double | Gets or sets the stroke size of the active area. |
Children | IList<IView> | Comment to avoid XmlDocCrash |
DragCompletedCommandParameter | object | Gets or sets the command parameter passed to DragCompletedCommand. If nothing is specified an empty instance of EventArgs is used. |
DragStartedCommandParameter | object | Gets or sets the command parameter passed to DragStartedCommand. If nothing is specified an empty instance of EventArgs is used. |
EndAngle | double | Gets or sets the ending angle where the active area will end. Angles are inverse clockwise. |
InactiveColor | Color | Gets or sets the color of the arc between StartAngle and EndAngle. |
InactiveStrokeLineCap | LineCap | Gets or sets the stroke cap of the inactive area. |
InactiveThickness | double | Gets or sets the stroke size of the inactive area. |
InnerBackgroundColor | Color | Gets or sets the color of circumference inside the track. |
MaxValue | double | Gets o sets the value that would represent the EndAngle. |
MinValue | double | Gets o sets the value that would represent the StartAngle. |
StartAngle | double | Gets or sets the starting angle where the active area will start. Angles are inverse clockwise. |
TextColor | Color | Gets or sets the font color of the value label. |
TextFontSize | double | Gets or sets the font size of the value label. |
ThumbControlTemplate | ControlTemplate | Gets or sets the thumb template. |
TrackColor | Color | Gets or sets the background circle color. |
Value | double | Gets o sets the current value. |
ValueChangedCommandParameter | object | Gets or sets the command parameter passed to ValueChangedCommand. If nothing is specified an instance of ValueChangedEventArgs is used. |
ValueFormat | string | Gets or sets the format to be applied to the value label. |
Events
Name | Type | Description |
---|---|---|
DragCompleted | EventHandler | Event invoked when the CircularSlide drag completes. |
DragStarted | EventHandler | Event invoked when the CircularSlide drag starts. |
ValueChanged | EventHandler<ValueChangedEventArgs> | Event invoked when the CircularSlide value changes. |
Commands
Name | Type | Description |
---|---|---|
DragCompletedCommand | ICommand | Gets or sets a command invoked when the CircularSlider drag completes. |
DragStartedCommand | ICommand | Gets or sets a command invoked when the CircularSlider drag starts. |
ValueChangedCommand | ICommand | Gets or sets a command that is invoked when the CircularSlider value changes. |