Legends
Legends are very important but it's not usually easy to customize how they look. To facilitate this, Grial provides separated controls that support templates and can be placed anywhere.
To associate the Chart and the Legend, the Legend
property of the Chart has to be set with the x:Name
of the Legend instance. Once you do that, all the data required to display the legend will be available in the Values
property of the Legend control.
There are 2 types of Legends: SingleSeriesLegend
and MultiSeriesLegend
.
SingleSeriesLegend
SingleSeriesLegend
is meant to be used with the PieChart
since that chart support just one series.
Definition
public partial class SingleSeriesLegend : ContentView, ILegend
Properties
Name | Type | Description | Default |
---|---|---|---|
Values | IEnumerable<ILegendSeries> | Gets or Sets the data that the legend will draw | null |
Series | ChartSeries | Gets or Sets the series from where Values collection was created | null |
ItemTemplate | DataTemplate | Gets or Sets the template of the items | null |
ItemsLayout | IItemsLayout | Gets or Sets the layout that items will have when drawn | null |
ILegendSeries Properties
Name | Type | Description |
---|---|---|
Source | object | Contains the object that was used when creating the data of the legend |
Text | string | The string representing the reference in the legend |
Color | Color | The color representing the reference in the legend |
The data in the Values
property is used to render the items. ILegendSeries
provides access to the Text
and Color
, which contains the actual color used to draw the value. It also has the specific item that was provided to the ItemsSource
of the Series
in case some extra data is required.
Xaml
<grial:SingleSeriesLegend x:Name="legend">
<grial:SingleSeriesLegend.ItemTemplate>
<DataTemplate>
<Grid
ColumnDefinitions="15,*,Auto"
Margin="0,5"
ColumnSpacing="6">
<Rectangle
Grid.Column="0"
Fill="{ Binding Color }"
HeightRequest="14"
HorizontalOptions="Center"
VerticalOptions="Center"
WidthRequest="14" />
<Label
Grid.Column="1"
FontSize="12"
Text="{ Binding Text }"
VerticalOptions="Center" />
<Label
Grid.Column="2"
FontAttributes="Bold"
FontSize="12"
Text="{Binding Source.Value}"
VerticalOptions="Center"
HorizontalOptions="End" />
<Rectangle
HeightRequest="2"
Fill="LightGray"
Grid.Column="1"
Margin="0,6,0,0"
Grid.ColumnSpan="2"
VerticalOptions="End"
/>
</Grid>
</DataTemplate>
</grial:SingleSeriesLegend.ItemTemplate>
</grial:SingleSeriesLegend>
Result
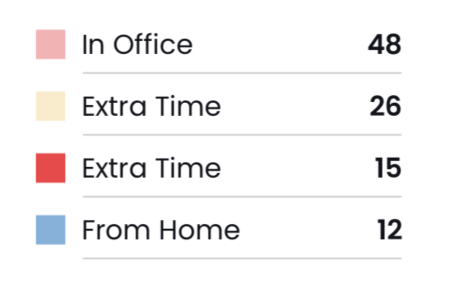
The actual Series
is also set in the SingleSeriesLegend
in case it's needed.
tip
If no template is provided, Grial uses an instance of LegendDefaultTemplate
to display the items to make sure something is always displayed.
MultiSeriesLegend
MultiSeriesLegend
is meant to be used with category charts since they support multiple series. Accessing the data is done in a similar fashion than in the SingleSeriesLegend
, with the exception that the Source
property of the ILegendSeries
has an entire series, instead of a single item.
Definition
public partial class MultiSeriesLegend : ContentView, ILegend
Properties
Name | Type | Description | Default |
---|---|---|---|
Values | IEnumerable<ILegendSeries> | Gets or Sets the data that the legend will draw | null |
ItemTemplate | DataTemplate | Gets or Sets the template of the items | null |
ItemsLayout | IItemsLayout | Gets or Sets the layout that items will have when drawn | null |